前置
- axios
- element-ui
表格的代码
<el-table :data="tableData" style="width: 100%">
<el-table-column prop="name" label="用户名"></el-table-column>
<el-table-column prop="email" label="邮箱"></el-table-column>
<el-table-column prop="address" label="地址"></el-table-column>
<el-table-column prop="string" label="星级"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button
size="mini"
type="danger"
>删除</el-button
>
<el-button
size="mini"
type="primary"
>编辑</el-button
>
</template>
</el-table-column>
</el-table>
表格data
data() {
return {
tableData: []
}
axios获取easymockjs的随机数据
methods: {
getuserslist() {
this.axios
.get("https://easy-mock.com/mock/5f8911f74dc90c6644514ed4/example/mock")
.then((res) => {
this.tableData = res.data.data.projects;
});
},
created初始化数据
created() {
this.getuserslist();
},
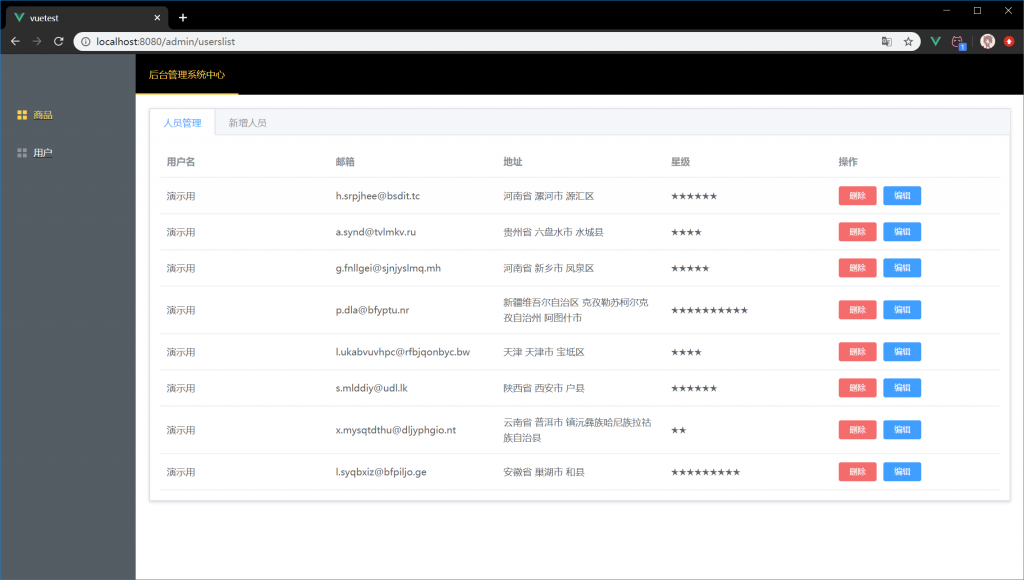
制作删除部分:
删除按钮绑定
@click.native.prevent="deleteRow(scope.$index, tableData)
方法:
deleteRow(index, tableData) {
tableData.splice(index, 1);
},
制作编辑部分
需要用到弹框来编辑,所以这里直接用element-ui的dialog
文档:https://element.eleme.cn/#/zh-CN/component/dialog
编辑按钮绑定
@click.native.prevent="openEditWindow(scope.$index, tableData)
dialog部分
<el-dialog title="编辑" :visible.sync="dialogFormVisible">
<el-form :model="form">
<el-form-item label="用户名" :label-width="formLabelWidth">
<el-input v-model="form.name" autocomplete="off" value=""></el-input>
</el-form-item>
<el-form-item label="邮箱" :label-width="formLabelWidth">
<el-input v-model="form.email" autocomplete="off"></el-input>
</el-form-item>
<el-form-item label="地址" :label-width="formLabelWidth">
<el-input v-model="form.address" autocomplete="off"></el-input>
</el-form-item>
<el-form-item label="星级" :label-width="formLabelWidth">
<el-input v-model="form.string" autocomplete="off"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogFormVisible = false">取 消</el-button>
<el-button type="primary" @click="subEdit">确 定</el-button>
</div>
</el-dialog>
方法:
//打开编辑窗口
openEditWindow(index, tableData) {
this.form.index = index;
this.form.name = tableData[index].name;
this.form.email = tableData[index].email;
this.form.address = tableData[index].address;
this.form.string = tableData[index].string;
this.dialogFormVisible = true;
},
//提交编辑
subEdit() {
this.dialogFormVisible = false;
this.editContent = {
name: this.form.name,
email: this.form.email,
address: this.form.address,
string: this.form.string,
};
this.tableData.splice(this.form.index, 1, this.editContent);
},
data部分
form: {
index: "",
name: "",
email: "",
address: "",
string: "",
},
dialogFormVisible: false,
editContent: "",
formLabelWidth: "100px",
效果
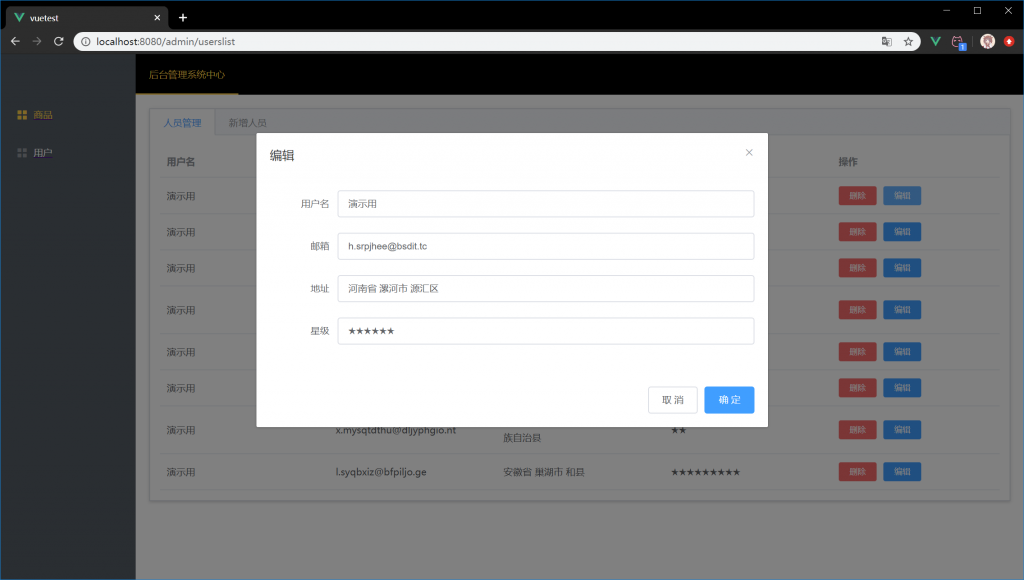
如需真提交修改subEdit部分即可,easymock我还没玩熟......
全部代码
<template>
<div>
<el-table :data="tableData" style="width: 100%">
<el-table-column prop="name" label="用户名"></el-table-column>
<el-table-column prop="email" label="邮箱"></el-table-column>
<el-table-column prop="address" label="地址"></el-table-column>
<el-table-column prop="string" label="星级"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button
size="mini"
@click.native.prevent="deleteRow(scope.$index, tableData)"
type="danger"
>删除</el-button
>
<el-button
size="mini"
@click.native.prevent="openEditWindow(scope.$index, tableData)"
type="primary"
>编辑</el-button
>
</template>
</el-table-column>
</el-table>
<el-dialog title="编辑" :visible.sync="dialogFormVisible">
<el-form :model="form">
<el-form-item label="用户名" :label-width="formLabelWidth">
<el-input v-model="form.name" autocomplete="off" value=""></el-input>
</el-form-item>
<el-form-item label="邮箱" :label-width="formLabelWidth">
<el-input v-model="form.email" autocomplete="off"></el-input>
</el-form-item>
<el-form-item label="地址" :label-width="formLabelWidth">
<el-input v-model="form.address" autocomplete="off"></el-input>
</el-form-item>
<el-form-item label="星级" :label-width="formLabelWidth">
<el-input v-model="form.string" autocomplete="off"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogFormVisible = false">取 消</el-button>
<el-button type="primary" @click="subEdit">确 定</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
form: {
index: "",
name: "",
email: "",
address: "",
string: "",
},
dialogFormVisible: false,
editContent: "",
formLabelWidth: "100px",
};
},
created() {
this.getuserslist();
},
methods: {
getuserslist() {
this.axios
.get("https://easy-mock.com/mock/5f8911f74dc90c6644514ed4/example/mock")
.then((res) => {
this.tableData = res.data.data.projects;
});
},
//打开编辑窗口
openEditWindow(index, tableData) {
this.form.index = index;
this.form.name = tableData[index].name;
this.form.email = tableData[index].email;
this.form.address = tableData[index].address;
this.form.string = tableData[index].string;
this.dialogFormVisible = true;
},
//提交编辑
subEdit() {
this.dialogFormVisible = false;
this.editContent = {
name: this.form.name,
email: this.form.email,
address: this.form.address,
string: this.form.string,
};
this.tableData.splice(this.form.index, 1, this.editContent);
},
deleteRow(index, tableData) {
tableData.splice(index, 1);
},
},
};
</script>